In this tutorial, you will clearly learn about how to transfer text using Arduino. Many think that as Arduino is not a wi-fi module we cannot transfer data from one system to the other. Transferring text is a building block of many projects where we need two or more devices to communicate with each other. It is one of the most common and useful techniques to send information or instructions from one system to another system. From the basic systems to IoT, transferring text is one of the most effective ways to check whether the system is working properly or not. Here, we are going to show you how you can send data of a DHT11 sensor from one Arduino to another using the ESP8266 wi-fi module. You will get the basic idea of how two devices can communicate using text.
Components Required
Hardware Required:
For this project, we are going to use two Arduino UNO. Next and most important module for this project, ESP8266 wifi module. We are going to use two ESP8266-01 modules. But you can use ESP8266-12E or 12F as well. Or you can replace both Arduino and wi-fi modules with two NodeMCU. Then we shall use a DHT11 temp and humidity sensor for data input and an LCD 16×2 display for data output. We shall also need a Potentiometer to control the contrast of the display. We may also need a separate 3.3V power source because sometimes 3.3V of Arduino can not supply enough current. Apart from this, we shall need a Breadboard and some Jumper wires.
Software Required:
Whether you are using Arduino or NodeMCU, you can always program them using Arduino IDE.
ESP8266-01 Wi-fi Module:
ESP8266-01 is an 8 pin connectivity module that allows microcontrollers to use wi-fi. It has 2 GPIO pins. It uses 3.3V as VCC and you need to be very careful while powering it. The value of VCC should not cross 3.3V. Next, we have TX and RX pins. This module is a transceiver, which means it can transmit as well as receive data. That is why we get both TX and RX pins. Using this module, you can directly connect with the internet using an IP address. You can also create a local hotspot using wi-fi and connect other wi-fi modules with it.

Downloading Libraries:
Whether you are using Arduino or NodeMCU, you can always program them using Arduino IDE. But for ESP8266 we use a library named “ESP8266WiFi.h” for our convenience. To use that library, we need to install some boards in our IDE. To install them, follow these instructions:
- Open the Arduino IDE and connect with the internet.
- Go to File → Preferences. Then paste the following link in the ‘Additional Board Manager URLs’ box.
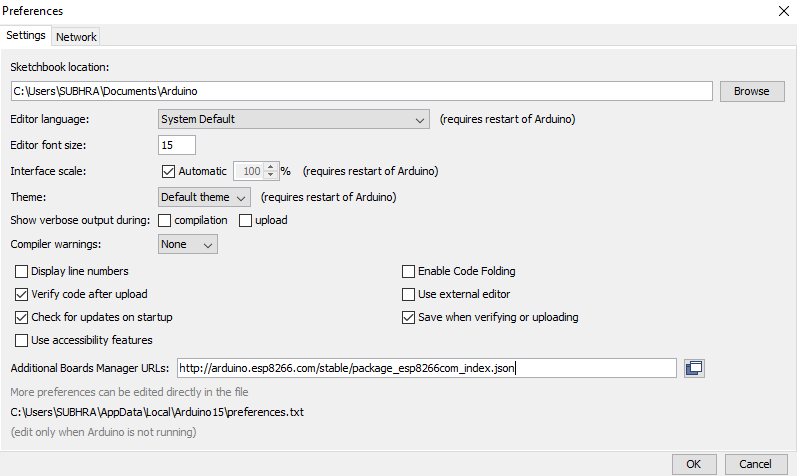
- Go to Tools → Board → Boards Manager…
- Then search esp8266 and install the following board.

- And again go to Tools → Board. Then scroll down and select Arduino. But if you want to use Node MCU, choose a suitable NodeMCU board.
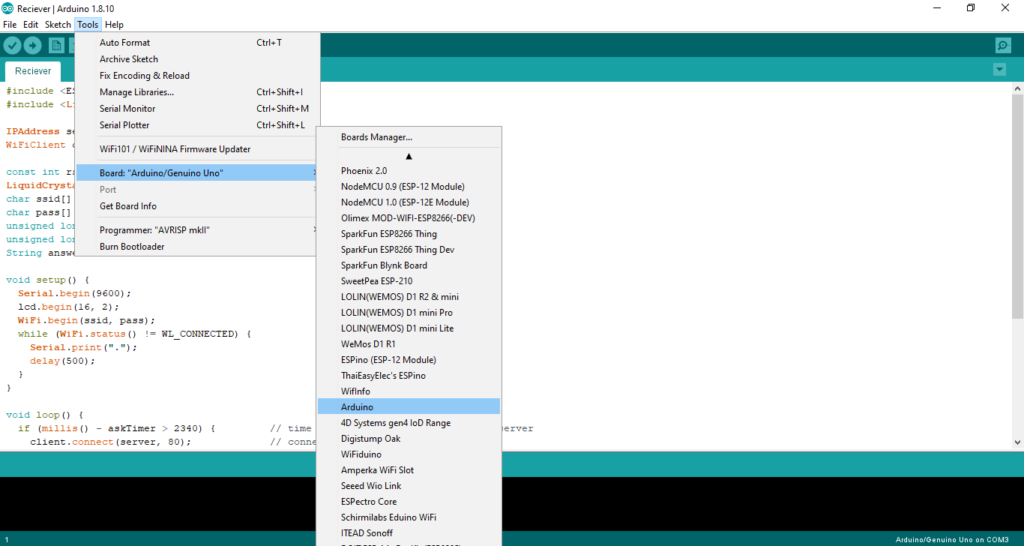
Apart from these boards and libraries, we’ll also need DHT.h and LiquidCrystal.h library. Check out our Arduino weather Station tutorial to know how to download the DHT.h library.
Circuit Diagrams with Connections:
- First let’s discuss the connection of ESP8266-01. We are using one Arduino as sender (wi-fi hotspot) and another as receiver. The connections for ESP8266-01 will be same for both.
- GND – GND of Arduino
- VCC – 3.3V
- TX – TX (Pin 1)
- RX – RX (Pin 0)
- En – 3.3V

- Then we connect the DHT11 sensor to the sender Arduino using the following connections
- VCC → +5V of Arduino
- GND → GND of Arduino
- DATA → Pin 2 of Arduino

- Next, we will connect the LCD with the receiver Arduino Pin D4, D5, D6, D7 of LCD are connected to the pin D5, D4, D3, D2 of Arduino respectively. The enable pin of LCD is connected to the pin D11 of Arduino. RS pin of LCD is connected to the D12 pin of Arduino. VSS and RW pins of LCD are connected to the ground. VDD pin is connected to 5V. VEE pin is connected to Potentiometer. VCC and GND pin is connected to 5V and ground respectively.
To summarize all these connections, we have given another circuit diagram with GND and VCC connections below. Though we connected VCC of ESP8266 with 3.3Vof Arduino, we suggest you connect with a different 3.3V power source.
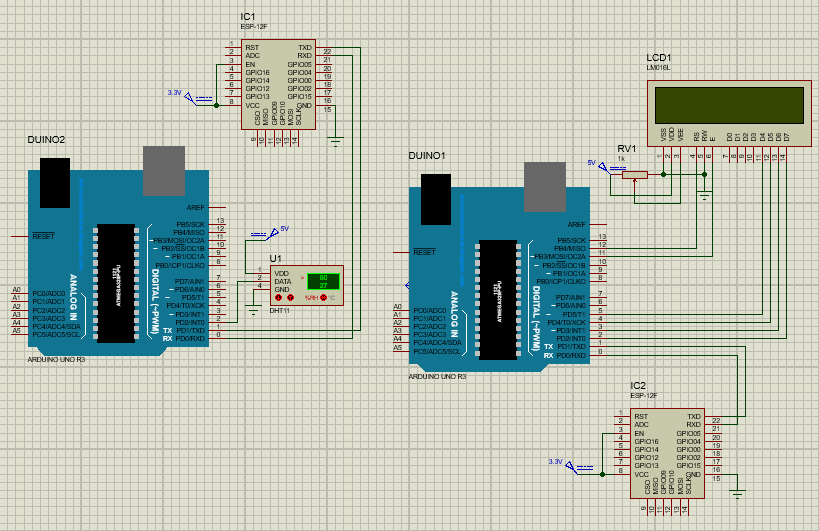
Code:
We have created two .ino files named Sender.ino and Reciever.ino.
Sender’s Code
#include "DHT.h"
#include <ESP8266WiFi.h>
#define DHTPIN 2
#define DHTTYPE DHT11
WiFiServer server(80); // launches the server
IPAddress ip(192, 168, 0, 80); // IP of the server
IPAddress gateway(192,168,0,1); // WiFi router's IP
IPAddress subnet(255,255,255,0);
DHT dht(DHTPIN, DHTTYPE);
char ssid[] = "Server";
char pass[] = "12345";
float h, t;
unsigned long DTimer = 0;
unsigned long CTimer = 0;
void setup(){
Serial.begin(9600);
dht.begin();
server_begin(0);
delay(2000);
}
void loop(){
if (millis() > DTimer + 2000) {
h = dht.readHumidity();
t = dht.readTemperature(); // reads the DHT for temperature
if (isnan(h) || isnan(t)) {
return;
} else {
DTimer = millis();
}
}
WiFiClient client = server.available();
if (client) {
if (client.connected()) {
client.flush();
client.println(String(t, 1)); // sends temp to the reciever
}
client.stop(); // reciever is disconnected
CTimer = millis();
}
if (millis() - CTimer > 30000) { // stops and restarts the WiFi server after 30 sec
WiFi.disconnect(); // idle time
delay(500);
server_begin(1);
}
}
void server_begin(byte trigger) {
if (trigger) {
Serial.print("Server Restart");
} else {
Serial.print("Server Start");
}
WiFi.config(ip, gateway, subnet);
WiFi.begin(ssid, pass);
while (WiFi.status() != WL_CONNECTED) {
Serial.print(".");
delay(500);
}
server.begin();
delay(500);
CTimer = millis();
}
Receiver’s Code
#include <ESP8266WiFi.h>
#include <LiquidCrystal.h>
IPAddress server(192,168,0,80); // fix IP of the server
WiFiClient client;
const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 2;
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
char ssid[] = "Server";
char pass[] = "12345";
unsigned long Timer = 0;
String data;
void setup() {
Serial.begin(9600);
lcd.begin(16, 2);
WiFi.begin(ssid, pass);
while (WiFi.status() != WL_CONNECTED) {
Serial.print(".");
delay(500);
}
}
void loop() {
if (millis()>Timer + 2000) { // time for each session
client.connect(server, 80); // connect to the server
data = client.readStringUntil('\r'); // received the server's data
client.flush();
Timer = millis();
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Temp:");
lcd.print(data);
}
}
Explanation of the Code:
Let’s first discuss sender.ino. At the beginning of the program we have included the <ESP8266WiFi.h> library. Then we have launched the server, fixed IP of the server and gateway, and fixed a subnet. We assigned a SSID which is the name of the network and password for security. Then in the setup() function, we have called server_begin(0) function which sets up the server.
Then in the loop() function, we have an if statement in the beginning. This sets up the time for which the data will be collected from the sensor. After that, we create a client which is the receiver. If it is connected to the sender then the sender will send the sensor data to the receiver for a fixed time and then disconnect it.
Now let’s discuss the receiver code. Here we have the same IP, SSID, and password as it was for the server. Then in the setup() function, the wi-fi has been set up. Next, in the loop() function in the if statement we have added a time for which the client will get data. And then it will read data at that time and print it to the display. We didn’t explain the DHT.h and LiquidCrytal.h library. For that please check our tutorial on Weather Station where you can clearly understand.
Finally, hope that you are clear in transferring data using Arduino. You can change the digital pins as of your convenience by modifying the pins in the code. If you face any problem please feel free to comment below and comment box is always open for you.